Intro
Master VBA nested for loops with this comprehensive guide, covering loop syntax, examples, and best practices for efficient coding, including nested loop structures and error handling techniques.
The world of VBA programming can be a complex and intimidating place, especially for those who are new to the concept of nested loops. However, with the right guidance and a clear understanding of the basics, anyone can master the art of using nested For loops in VBA. In this article, we will delve into the importance of nested loops, their benefits, and provide a comprehensive guide on how to use them effectively in your VBA programming journey.
VBA, or Visual Basic for Applications, is a programming language developed by Microsoft that is widely used for creating and automating tasks in Microsoft Office applications such as Excel, Word, and Access. One of the most powerful tools in VBA programming is the For loop, which allows you to execute a block of code repeatedly for a specified number of times. However, when you need to perform a task that involves iterating over multiple sets of data or performing a task that requires multiple loops, nested For loops become essential.
Nested For loops are used when you need to execute a loop inside another loop. The inner loop will execute its entire cycle for each iteration of the outer loop, allowing you to perform complex tasks that involve multiple iterations. For example, if you need to print out the coordinates of a grid, you would use a nested loop to iterate over the rows and columns of the grid.
Understanding Nested For Loops
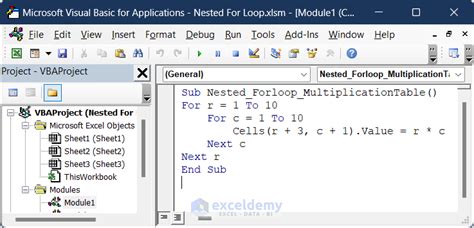
To understand how nested For loops work, let's consider a simple example. Suppose we want to print out the numbers 1 to 5, and for each number, we want to print out the letters A to E. We can achieve this using a nested For loop. The outer loop will iterate over the numbers, and the inner loop will iterate over the letters.
Example Code
```vb Sub NestedForLoopExample() Dim i As Integer Dim j As IntegerFor i = 1 To 5
For j = 1 To 5
Debug.Print i & " - " & Chr(64 + j)
Next j
Next i
End Sub
In this example, the outer loop iterates over the numbers 1 to 5, and the inner loop iterates over the numbers 1 to 5, printing out the corresponding letter using the `Chr` function.
Benefits of Nested For Loops
Nested For loops offer several benefits, including:
* **Improved code readability**: By using nested loops, you can break down complex tasks into smaller, more manageable chunks, making your code easier to read and understand.
* **Increased flexibility**: Nested loops allow you to perform complex tasks that involve multiple iterations, making them ideal for tasks such as data processing and analysis.
* **Reduced code duplication**: By using nested loops, you can avoid duplicating code, making your programs more efficient and easier to maintain.
Best Practices for Using Nested For Loops
To get the most out of nested For loops, follow these best practices:
* **Keep it simple**: Avoid using too many nested loops, as this can make your code difficult to read and understand.
* **Use meaningful variable names**: Use descriptive variable names to make your code easier to read and understand.
* **Test your code**: Always test your code to ensure it works as expected.
Common Pitfalls to Avoid
When using nested For loops, there are several common pitfalls to avoid, including:
* **Infinite loops**: Make sure your loops have a clear termination condition to avoid infinite loops.
* **Loop variable scope**: Be aware of the scope of your loop variables to avoid conflicts and unexpected behavior.
* **Performance issues**: Avoid using too many nested loops, as this can impact performance.
Debugging Nested For Loops
Debugging nested For loops can be challenging, but there are several techniques you can use to make the process easier, including:
* **Using the debugger**: Use the built-in debugger to step through your code and identify issues.
* **Printing debug output**: Use `Debug.Print` statements to print out debug information and track the flow of your program.
* **Using breakpoints**: Set breakpoints to pause execution and inspect variables and expressions.
Real-World Applications of Nested For Loops
Nested For loops have a wide range of real-world applications, including:
* **Data processing and analysis**: Nested loops are ideal for tasks such as data processing and analysis, where you need to iterate over multiple sets of data.
* **Game development**: Nested loops are used extensively in game development to create complex game logic and simulate real-world scenarios.
* **Scientific simulations**: Nested loops are used in scientific simulations to model complex systems and simulate real-world phenomena.
Example Use Cases
Here are a few example use cases for nested For loops:
* **Printing a grid**: Use a nested loop to print out the coordinates of a grid.
* **Simulating a game**: Use a nested loop to simulate a game, such as a game of chess or checkers.
* **Analyzing data**: Use a nested loop to analyze data, such as a set of sales figures or customer demographics.
Nested For Loop Gallery
What is a nested For loop?
+
A nested For loop is a loop that is inside another loop. The inner loop will execute its entire cycle for each iteration of the outer loop.
How do I use a nested For loop in VBA?
+
To use a nested For loop in VBA, you need to define the outer loop and the inner loop, and make sure the inner loop is fully executed for each iteration of the outer loop.
What are the benefits of using nested For loops?
+
The benefits of using nested For loops include improved code readability, increased flexibility, and reduced code duplication.
How do I debug a nested For loop?
+
To debug a nested For loop, you can use the built-in debugger, print debug output, and set breakpoints to pause execution and inspect variables and expressions.
What are some common pitfalls to avoid when using nested For loops?
+
Some common pitfalls to avoid when using nested For loops include infinite loops, loop variable scope issues, and performance issues.
In conclusion, nested For loops are a powerful tool in VBA programming that can help you perform complex tasks and improve the efficiency of your code. By following the best practices and avoiding common pitfalls, you can master the art of using nested For loops and take your VBA programming skills to the next level. Whether you're a beginner or an experienced programmer, this guide has provided you with the knowledge and skills you need to succeed. So, go ahead and start using nested For loops in your VBA programming today and see the difference for yourself. We invite you to share your experiences, ask questions, and provide feedback in the comments section below.