Intro
Learn VBA search techniques to find values in columns, using Excel VBA macros with efficient search methods and related functions like Range.Find and WorksheetFunction.Match.
The importance of searching for specific values within large datasets cannot be overstated, especially in Excel where data analysis is a core function. Visual Basic for Applications (VBA) provides a powerful toolset for automating tasks in Excel, including searching for values in columns. This capability is crucial for data manipulation, analysis, and reporting, allowing users to automate repetitive tasks and improve productivity. Whether you're a beginner or an advanced user, mastering VBA search functions can significantly enhance your workflow.
Searching for values in columns is a fundamental operation that can be applied in various contexts, from simple data retrieval to complex data analysis. For instance, in a sales database, you might need to find all entries for a specific product or customer, or in a financial spreadsheet, you might want to locate transactions above a certain threshold. The ability to efficiently search through data enables quicker decision-making and more accurate analysis.
The process of searching for values in columns using VBA involves several steps, including setting up your Excel sheet, writing the VBA code, and executing it. VBA's flexibility allows for different search methods, such as searching for exact matches, partial matches, or even searching across multiple columns and worksheets. This versatility makes VBA an indispensable tool for anyone working with data in Excel.
Introduction to VBA Search Functions
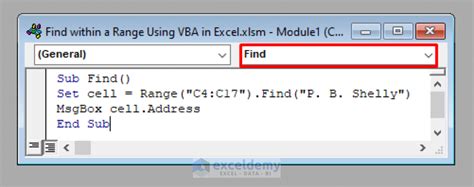
VBA search functions are designed to locate specific data within Excel worksheets. The most common method involves using the Range.Find
method, which allows for precise searches based on various criteria, including the search value, look-in range, look-at parameter, search order, and more. Understanding how to utilize these parameters effectively is key to performing successful searches.
Understanding the Range.Find Method
The `Range.Find` method is a powerful tool that enables detailed searches. It requires several arguments to be specified, such as `What`, `LookIn`, `LookAt`, `SearchOrder`, `SearchDirection`, `MatchCase`, and `MatchByte`. Each of these arguments plays a crucial role in defining the search parameters, from what value to search for to whether the search should be case-sensitive.Implementing VBA Search Functions

Implementing VBA search functions begins with opening the Visual Basic Editor in Excel, which can be done by pressing Alt + F11
or navigating to Developer > Visual Basic. Once in the editor, you can insert a new module to write your VBA code. A basic search function might look something like this:
Sub SearchValue()
Dim rng As Range
Set rng = Worksheets("Sheet1").Range("A1:A100").Find(What:="SearchValue", LookIn:=xlValues)
If Not rng Is Nothing Then
MsgBox "Value found in cell " & rng.Address
Else
MsgBox "Value not found"
End If
End Sub
This example searches for the value "SearchValue" in the range A1:A100 of Sheet1 and displays a message box indicating whether the value was found and its location.
Advanced Search Techniques
Beyond basic searches, VBA offers advanced techniques for more complex data retrieval tasks. This includes searching for partial matches using wildcard characters (`*` and `?`), searching across multiple columns or worksheets, and even using regular expressions for pattern matching. These advanced techniques significantly expand the capabilities of VBA search functions, allowing for more nuanced and sophisticated data analysis.Practical Applications of VBA Search
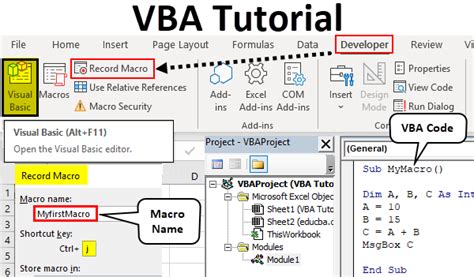
The practical applications of VBA search functions are vast and varied. In business, they can be used to automate tasks such as data cleaning, report generation, and dashboard updates. In research, they can facilitate data analysis by quickly identifying trends, patterns, and correlations within large datasets. For personal finance, VBA searches can help in tracking expenses, categorizing transactions, and setting budget alerts.
Best Practices for VBA Search
To get the most out of VBA search functions, it's essential to follow best practices. This includes optimizing your code for performance, using clear and descriptive variable names, and thoroughly testing your searches to ensure accuracy. Additionally, considering factors like data type, search scope, and potential errors can help in designing robust and reliable search functions.Gallery of VBA Search Examples
VBA Search Examples Gallery
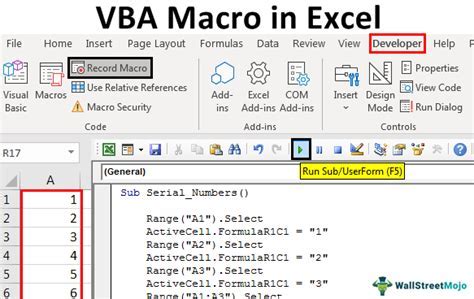
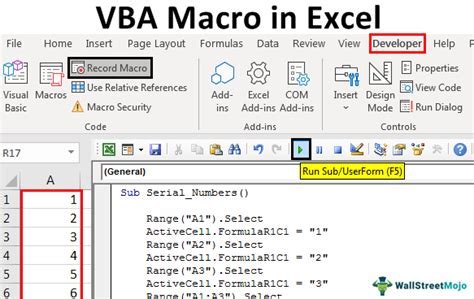
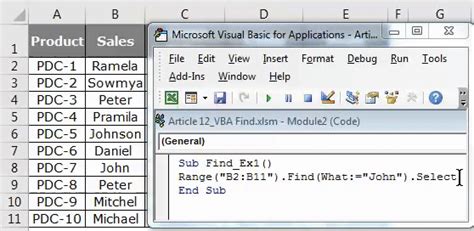
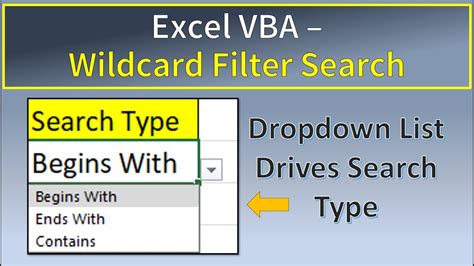
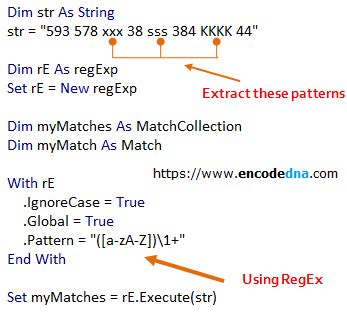
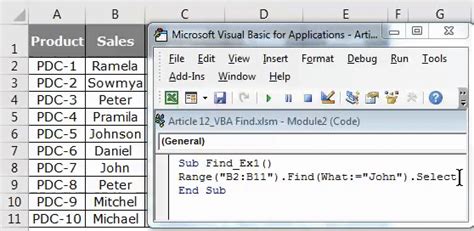
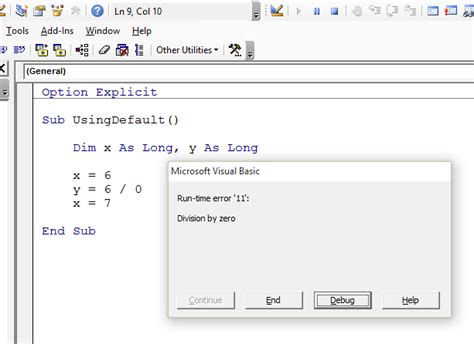
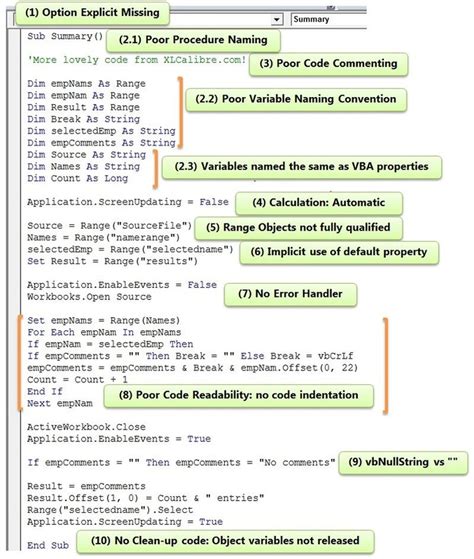
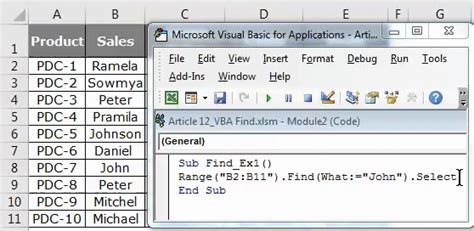
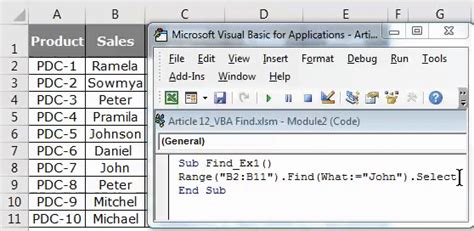
Frequently Asked Questions
What is the most efficient way to search for values in Excel using VBA?
+The most efficient way often involves using the `Range.Find` method, as it allows for direct and flexible searches. Optimizing the search range and parameters can significantly improve performance.
How can I search for partial matches in VBA?
+You can use wildcard characters (`*` and `?`) within the `What` argument of the `Find` method to search for partial matches. For example, `What:="*SearchValue*"` would find any cell containing "SearchValue" anywhere in its value.
Can VBA search functions be used across multiple worksheets or workbooks?
+Yes, VBA can be used to search across multiple worksheets or even workbooks. This involves looping through each worksheet or workbook and applying the search function to each range of interest.
In conclusion, mastering VBA search functions can revolutionize the way you work with data in Excel. Whether you're automating simple tasks or performing complex data analysis, the ability to efficiently search for and manipulate data is indispensable. By understanding the basics of VBA search, exploring advanced techniques, and applying best practices, you can unlock the full potential of Excel and take your data analysis skills to the next level. We invite you to share your experiences with VBA search functions, ask questions, or explore more topics related to Excel and VBA programming. Your feedback and engagement are invaluable in helping us create more informative and useful content for our readers.