Intro
Master Excel VBA with 5 ways to copy range, including cell referencing, loop iterations, and worksheet interactions, to boost productivity and automate tasks efficiently.
The ability to copy a range in Excel VBA is a fundamental skill for any aspiring Excel programmer. Copying ranges is essential for tasks such as data manipulation, report generation, and automation of repetitive tasks. Excel VBA provides several methods to achieve this, each with its own set of advantages and use cases. Here, we will explore five ways to copy a range in Excel VBA, discussing the benefits and applications of each method.
When working with Excel VBA, understanding the different methods for copying ranges can significantly enhance your productivity and the efficiency of your macros. Whether you are a beginner looking to automate simple tasks or an advanced user seeking to optimize complex workflows, mastering range copying techniques is crucial.
Copying ranges in Excel VBA can be approached in various ways, including using the Range.Copy
method, looping through cells, using arrays, employing the Range.AutoFill
method, and utilizing the Worksheet_Paste
event. Each of these methods has its unique characteristics and is suited for different scenarios.
Understanding the Basics of Range Copying in Excel VBA
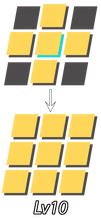
Before diving into the specific methods, it's essential to understand the basics of working with ranges in Excel VBA. A range in Excel refers to a cell or a group of cells. Excel VBA allows you to manipulate these ranges in various ways, including copying their contents, formats, or both. The Range
object is central to these operations, and its properties and methods, such as Value
, Formula
, Font
, and Copy
, are frequently used.
Method 1: Using the Range.Copy Method
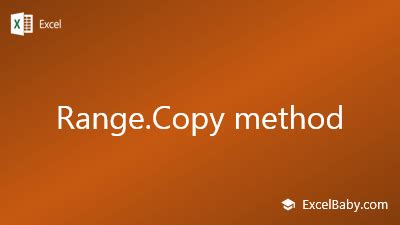
The most straightforward way to copy a range in Excel VBA is by using the Range.Copy
method. This method copies the entire range, including its formatting and formulas, to the clipboard. After executing the Copy
method, you can paste the range using the Range.Paste
method or manually through Excel's user interface.
Sub CopyRangeUsingCopyMethod()
Range("A1:B2").Copy Range("C1")
End Sub
This example copies the range A1:B2 and pastes it starting from cell C1. The Copy
method is convenient but can be slow for large datasets due to the involvement of the clipboard.
Method 2: Looping Through Cells
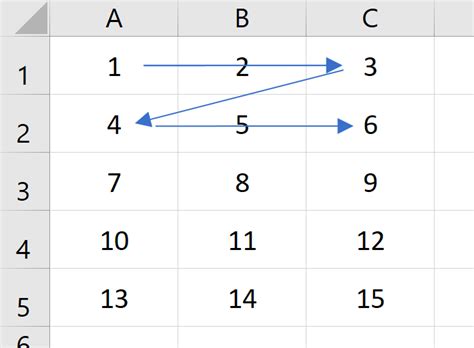
Another approach is to loop through each cell in the source range and manually copy its value or formatting to the destination range. This method provides fine-grained control over what is copied and can be more efficient for certain operations.
Sub CopyRangeByLooping()
Dim sourceRange As Range
Dim targetRange As Range
Dim i As Long, j As Long
Set sourceRange = Range("A1:B2")
Set targetRange = Range("C1")
For i = 1 To sourceRange.Rows.Count
For j = 1 To sourceRange.Columns.Count
targetRange.Offset(i - 1, j - 1).Value = sourceRange.Cells(i, j).Value
Next j
Next i
End Sub
This example demonstrates how to copy values from one range to another by looping through cells. It's more flexible than the Copy
method but can be slower for large ranges.
Method 3: Using Arrays
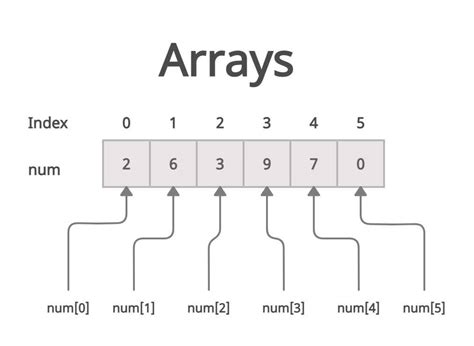
Utilizing arrays is a highly efficient method for copying ranges, especially when dealing with large datasets. By assigning the range's values to an array, manipulating the array, and then assigning it back to a range, you can bypass the clipboard and improve performance.
Sub CopyRangeUsingArrays()
Dim sourceArray As Variant
Dim targetRange As Range
sourceArray = Range("A1:B2").Value
Set targetRange = Range("C1")
targetRange.Resize(UBound(sourceArray, 1), UBound(sourceArray, 2)).Value = sourceArray
End Sub
This method is not only fast but also allows for easy manipulation of the data before it's written back to the worksheet.
Method 4: Using the Range.AutoFill Method
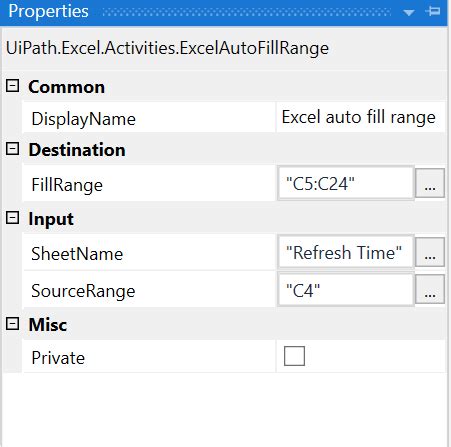
The AutoFill
method is useful for copying formulas or patterns down to a larger range. It automatically fills the target range with the formula or pattern from the source range.
Sub CopyRangeUsingAutoFill()
Range("A1").AutoFill Destination:=Range("A1:A10")
End Sub
This example copies the formula or value in cell A1 down to cells A2 through A10.
Method 5: Utilizing the Worksheet_Paste Event
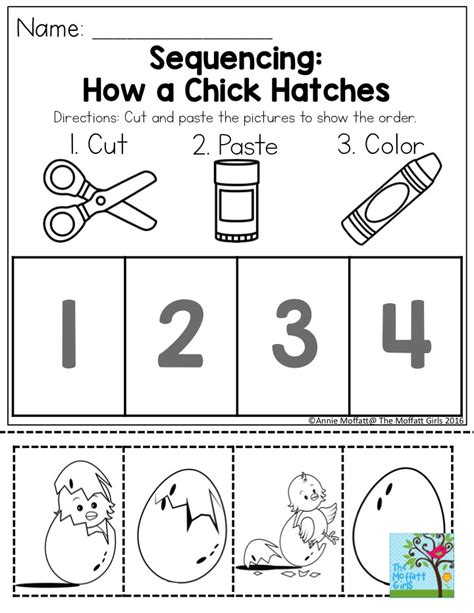
Although not a direct method for copying ranges, the Worksheet_Paste
event can be used to intercept and manipulate paste operations within a worksheet. This can be particularly useful for enforcing formatting standards or handling data validation upon paste.
Private Sub Worksheet_Paste(ByVal Target As Range)
' Code to manipulate the pasted range
Target.Font.Size = 12
End Sub
This example changes the font size of the pasted range to 12 points.
Gallery of Excel VBA Copy Range Methods
Excel VBA Copy Range Methods Gallery
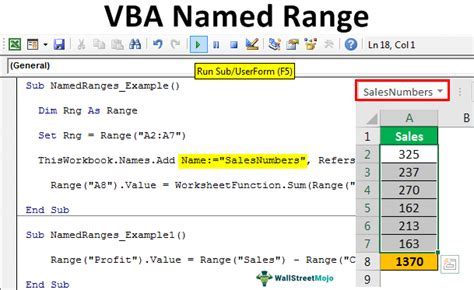
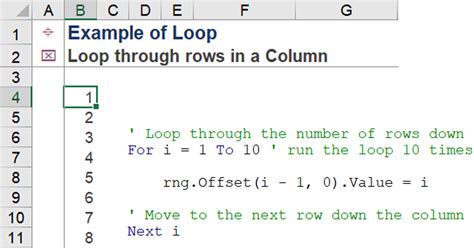
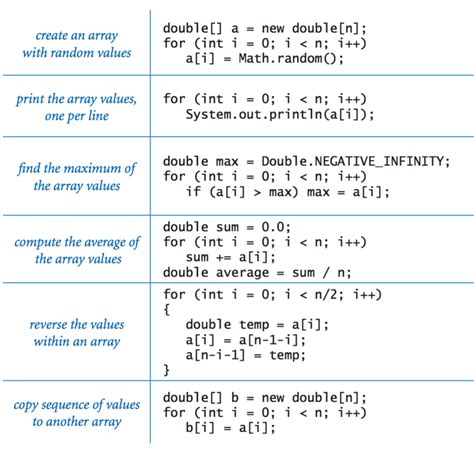
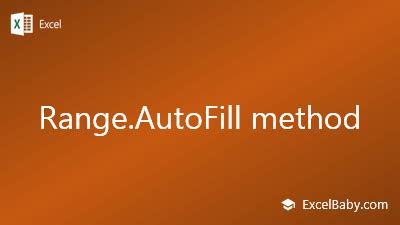
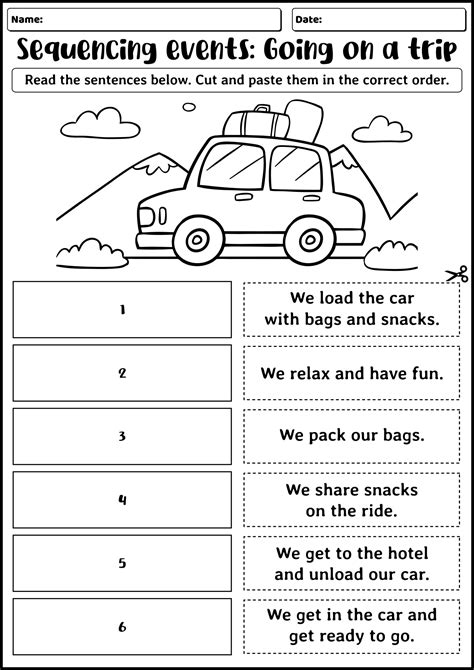
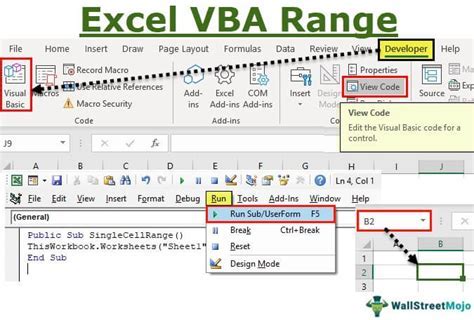
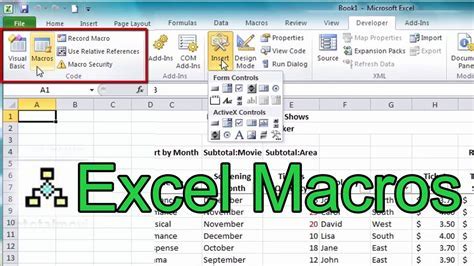
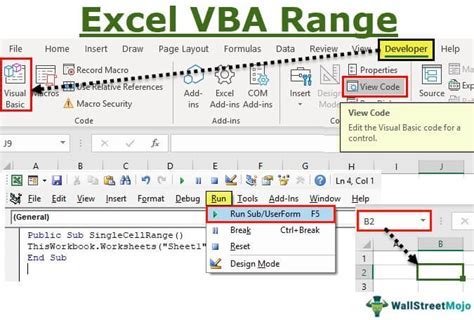


What is the fastest way to copy a range in Excel VBA?
+Using arrays is generally the fastest method for copying ranges in Excel VBA, especially for large datasets, as it bypasses the clipboard and directly manipulates the data in memory.
How do I copy a range with formatting in Excel VBA?
+To copy a range with formatting, you can use the `Range.Copy` method followed by `Range.PasteSpecial` with the `xlPasteFormats` argument to paste only the formats.
Can I use Excel VBA to copy a range to another workbook?
+Yes, you can use Excel VBA to copy a range to another workbook by activating the target workbook and worksheet, then using the `Range.Copy` method or other copying techniques mentioned.
In conclusion, mastering the various methods for copying ranges in Excel VBA is essential for efficient data manipulation and automation. By understanding the strengths and weaknesses of each method, you can choose the most appropriate technique for your specific needs, enhancing your productivity and the effectiveness of your macros. Whether you're working with small datasets or large, complex spreadsheets, the ability to copy ranges accurately and efficiently is a fundamental skill that will serve you well in your Excel VBA journey. We invite you to share your experiences, ask questions, or provide feedback on the methods discussed, helping to create a community that learns and grows together.