Intro
Discover 5 ways to remove left characters, including trimming whitespace, using string functions, and regex techniques to optimize text data, improve formatting, and enhance data cleansing and processing efficiency.
Removing left characters from a string can be a common requirement in various programming and data manipulation tasks. Whether you're working with text files, databases, or any other form of data, knowing how to efficiently trim or remove unwanted characters from the beginning of a string is essential. Here are five ways to remove left characters, each applicable in different contexts or programming languages.
The importance of mastering string manipulation techniques, including removing left characters, cannot be overstated. It's a fundamental skill for anyone working with data, whether in the context of web development, data analysis, or software engineering. The ability to clean and preprocess data effectively can significantly impact the efficiency and accuracy of subsequent operations, such as data analysis, pattern recognition, or simply presenting the data in a user-friendly format.
In many real-world scenarios, data is not always clean or properly formatted. For instance, when importing data from external sources, you might encounter strings with leading spaces, tabs, or other unwanted characters that need to be removed. Knowing how to remove these characters efficiently can save a lot of time and prevent potential errors down the line. Moreover, in programming, being able to manipulate strings with precision is crucial for tasks such as data validation, where removing left characters might be necessary to ensure that user input conforms to expected formats.
Understanding the Need for Removing Left Characters
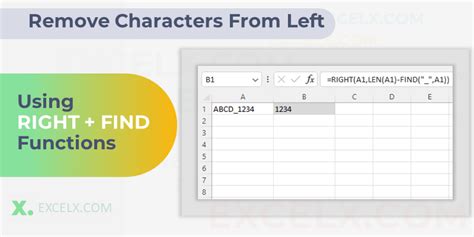
Before diving into the methods for removing left characters, it's essential to understand the context in which this operation is performed. In text processing, the term "left characters" refers to characters at the beginning of a string. Removing these characters can be necessary for various reasons, such as cleaning up data, preparing strings for comparison, or ensuring that data conforms to specific formatting requirements.
Method 1: Using String Functions in Programming Languages
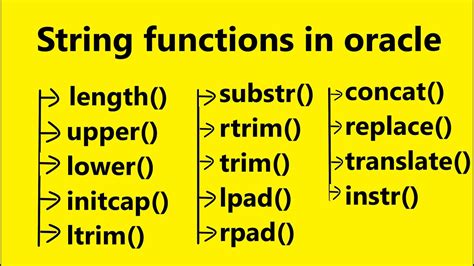
Most programming languages provide built-in string functions that can be used to remove left characters. For example, in Python, you can use the lstrip()
method, which removes leading characters (space is default) from the string. Here's a simple example:
my_string = " Hello World!"
my_string = my_string.lstrip()
print(my_string)
would output"Hello World!"
Similarly, in JavaScript, you can use the trimStart()
method to achieve the same result:
let myString = " Hello World!";
myString = myString.trimStart();
console.log(myString)
would output"Hello World!"
Steps for Using String Functions
1. Identify the string from which you want to remove left characters. 2. Choose the appropriate string function based on your programming language (e.g., `lstrip()` in Python, `trimStart()` in JavaScript). 3. Apply the function to your string, optionally specifying the characters you want to remove if the function allows for it. 4. Assign the result back to a variable or use it directly in your code.Method 2: Regular Expressions
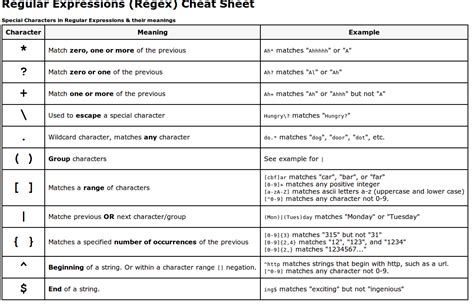
Regular expressions (regex) provide a powerful way to match and manipulate patterns in strings, including removing left characters. The pattern ^
matches the start of a string, and by combining it with other patterns, you can specify what characters to remove from the beginning of a string.
For example, in Python, you can use the re.sub()
function from the re
module:
import re
my_string = "###Hello World!"
my_string = re.sub('^#*', '', my_string)
print(my_string)
would output"Hello World!"
In JavaScript, you can use the replace()
method with a regex pattern:
let myString = "###Hello World!";
myString = myString.replace(/^#*/, '');
console.log(myString)
would output"Hello World!"
Benefits of Using Regular Expressions
- Flexibility: Regex patterns can be tailored to match specific characters or patterns at the start of a string. - Power: Regex can handle complex string manipulation tasks beyond simple character removal. - Universality: Regex is supported in most programming languages, making it a versatile tool for string manipulation.Method 3: Manual Looping
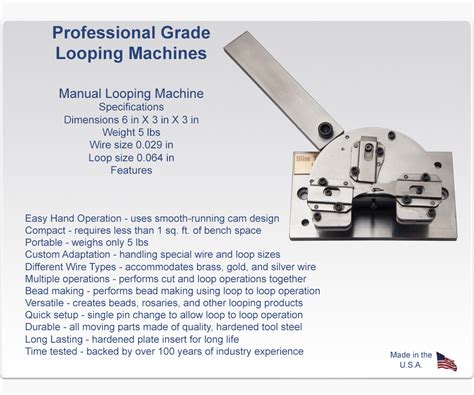
In some cases, especially when working with very specific requirements or in environments where built-in string functions are limited, manually looping through the characters of a string can be an effective way to remove left characters. This method involves iterating through the string from the beginning, checking each character against a condition (e.g., whether it's a space or a specific character), and appending characters that do not match the condition to a new string.
Here's an example in Python:
my_string = " Hello World!"
new_string = ""
started = False
for char in my_string:
if not started and char == " ":
continue
else:
started = True
new_string += char
print(new_string)
would output"Hello World!"
And in JavaScript:
let myString = " Hello World!";
let newString = '';
let started = false;
for (let char of myString) {
if (!started && char === ' ') {
continue;
} else {
started = true;
newString += char;
}
console.log(newString)
would output"Hello World!"
Considerations for Manual Looping
- Efficiency: Manual looping can be less efficient than using built-in functions, especially for large strings. - Complexity: This method requires more code and can be more prone to errors if not implemented carefully.Method 4: Using Substring
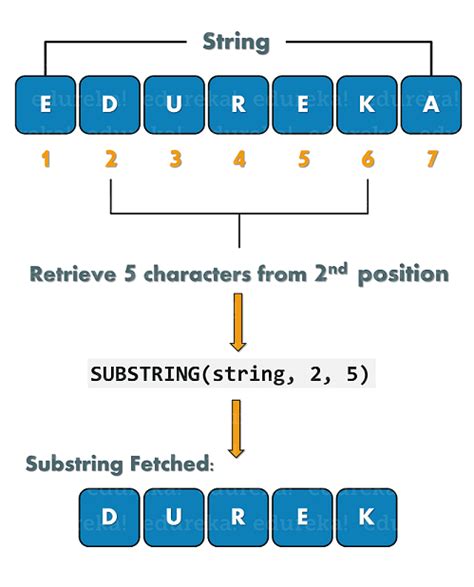
Another approach to removing left characters is by using the substring method, which allows you to extract a portion of a string. By starting the substring from the position right after the characters you want to remove, you can effectively remove those characters.
For example, if you know that the first n
characters are the ones you want to remove, you can use the following approach in Python:
my_string = " Hello World!"
my_string = my_string[3:]
(assuming the first 3 characters are the ones to remove)print(my_string)
would output"Hello World!"
Similarly, in JavaScript:
let myString = " Hello World!";
myString = myString.substring(3);
(again, assuming the first 3 characters are to be removed)console.log(myString)
would output"Hello World!"
Steps for Using Substring
1. Determine the number of characters to remove from the start. 2. Use the substring method to create a new string that starts after those characters. 3. Assign the result back to your variable or use it directly.Method 5: String Split and Join
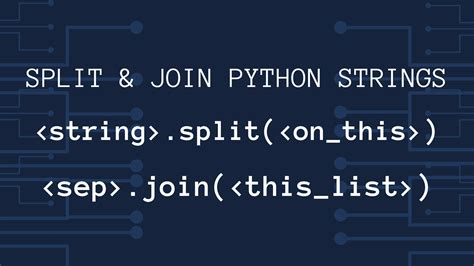
This method involves splitting the string into parts based on the character you want to remove and then joining the parts back together, excluding the unwanted characters from the beginning. It's more commonly used when the characters to be removed are not just spaces but can be any specific character or set of characters.
For instance, in Python, if you want to remove all occurrences of a specific character from the start of a string, you could split the string by that character and then join the resulting list of strings, starting from the first non-empty string:
my_string = "###Hello World!"
parts = my_string.split('#')
my_string = ''.join([part for part in parts if part])
print(my_string)
would output"Hello World!"
In JavaScript, a similar approach can be taken:
let myString = "###Hello World!";
let parts = myString.split('#');
myString = parts.filter(part => part).join('');
console.log(myString)
would output"Hello World!"
Advantages of String Split and Join
- Flexibility: This method allows for easy removal of specific characters from the start of a string. - Readability: The code can be quite readable, especially when working with arrays and string methods.Remove Left Characters Image Gallery
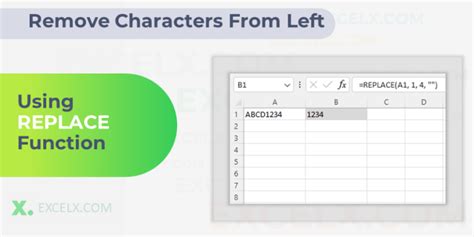
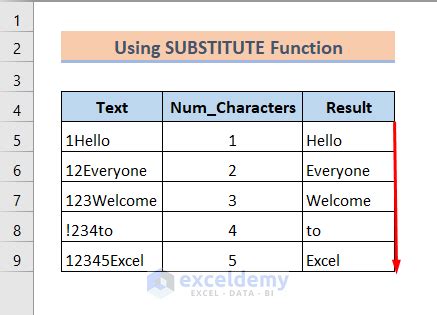
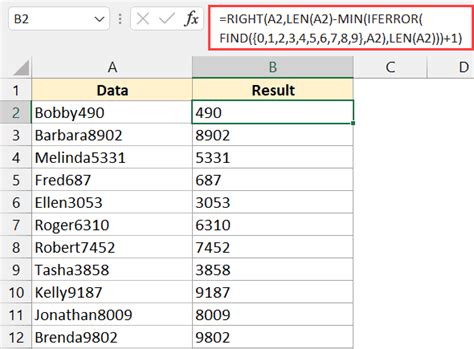
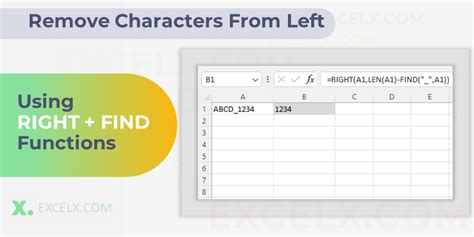
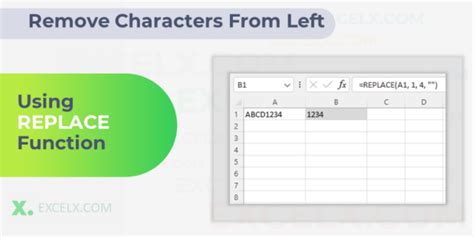
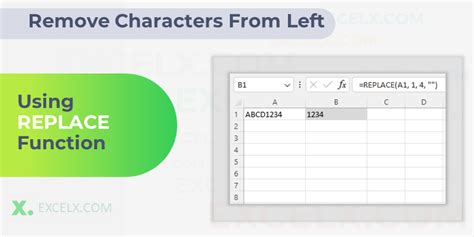
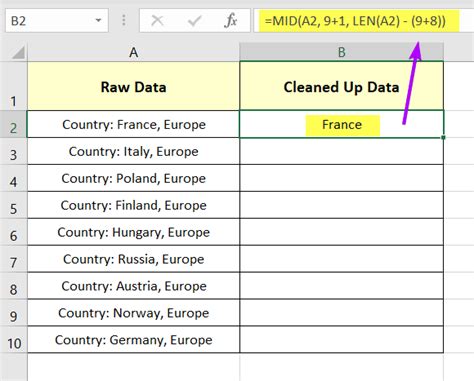

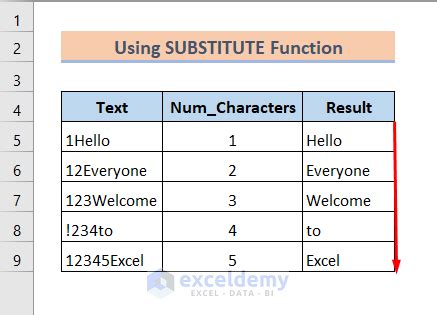
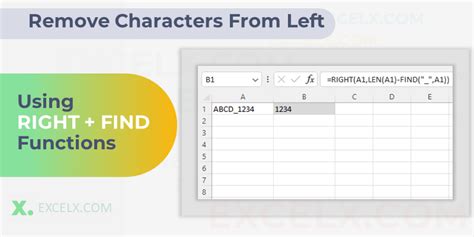
What are the most common reasons for removing left characters from a string?
+The most common reasons include cleaning up data, preparing strings for comparison, and ensuring that data conforms to specific formatting requirements.
How do I choose the best method for removing left characters in my specific situation?
+The choice of method depends on the programming language you're using, the nature of the characters you want to remove, and personal preference. Built-in string functions are usually the most efficient, but regular expressions offer flexibility for complex patterns.
Can I use these methods for removing characters from the end of a string as well?
+Yes, many of the methods described (such as using string functions or regular expressions) can be adapted to remove characters from the end of a string by adjusting the parameters or patterns used.
In conclusion, the ability to remove left characters from strings is a fundamental skill in programming and data manipulation. Whether through built-in string functions, regular expressions, manual looping, substring methods, or string split and join techniques, there are multiple approaches to achieve this, each with its own advantages and suitable use cases. By understanding and mastering these methods, developers and data analysts can efficiently preprocess and clean their data, paving the way for more accurate analysis, smoother program execution, and better decision-making. We invite you to share your experiences with removing left characters and any unique methods you've found particularly useful in your work. Your insights can help others facing similar challenges, and together, we can build a more comprehensive understanding of string manipulation techniques.