Intro
Discover 5 ways to append character in various programming languages, including string manipulation, concatenation, and formatting techniques, to enhance your coding skills and efficiently add characters to strings, texts, and data sets.
In the realm of computing and programming, appending characters to strings is a fundamental operation that can be achieved through various methods, depending on the programming language or context in which you are working. This operation is crucial for building dynamic strings, modifying existing text, or concatenating different pieces of information. Here, we will explore five common ways to append characters, discussing the approaches in a general sense and providing examples in popular programming languages like Python, JavaScript, and C++.
The importance of understanding how to append characters lies in its universal application across different programming tasks, from web development to system programming. Whether you are creating a simple script or a complex application, the ability to manipulate strings efficiently is indispensable. Moreover, mastering string manipulation can significantly improve your coding skills, making you more versatile and adept at handling a wide range of programming challenges.
Applying string concatenation or appending characters is not limited to programming. In data processing, text editing, and even in certain mathematical operations, understanding how to combine or modify strings is essential. This operation can be used to format data, create reports, or simply to modify existing text documents. Given its broad applicability, it's beneficial to grasp the fundamental concepts and methods of appending characters, which can then be applied across various domains and programming languages.
Using Concatenation Operators
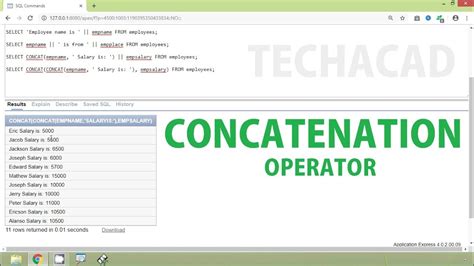
One of the most straightforward methods to append characters is by using concatenation operators. In many programming languages, such as Python, JavaScript, and Java, the "+" symbol is used for this purpose. For example, in Python, you can append a character to a string using the "+" operator like this: my_string = "Hello"; my_string += " World"
. This results in my_string
being "Hello World".
Utilizing String Formatting
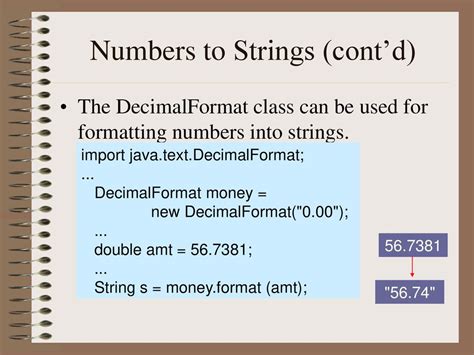
String formatting provides another versatile method for appending characters. This can be achieved through various techniques, including the use of format strings, f-strings (in Python), or template literals (in JavaScript). For instance, in Python, you can use an f-string to append a character to a string like this: character = 'A'; my_string = f"Hello {character}"
, resulting in "Hello A".
String Interpolation
String interpolation is a feature of some programming languages that allows you to embed expressions inside string literals. This can be a powerful way to append characters or variables to strings. In JavaScript, for example, template literals allow you to embed expressions inside template strings, using the ${expression}
syntax.
Employing StringBuilder or Equivalent
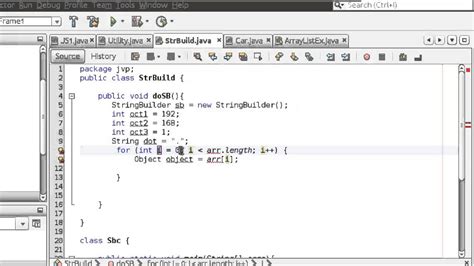
In languages like Java or C#, the StringBuilder
class (or its equivalent) is designed for efficient string manipulation, including appending characters. This approach is particularly useful when dealing with a large number of string concatenations, as it can improve performance by reducing the number of temporary strings created.
Regular Expressions and String Manipulation Functions
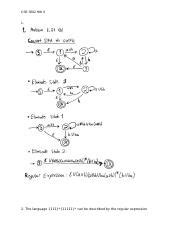
Regular expressions offer a powerful way to search, validate, and extract data from strings, and can also be used to append characters under certain conditions. Additionally, many programming languages provide built-in string manipulation functions, such as substr
, concat
, or replace
, which can be used to append characters or modify strings in various ways.
Practical Examples and Advice
When appending characters, it's essential to consider the performance implications, especially in loops or when dealing with large datasets. Some methods, like using the "+" operator in a loop, can be inefficient due to the creation of temporary strings. In such cases, using a StringBuilder
or an array to accumulate the characters and then converting it to a string can be more efficient.
Gallery of String Manipulation Techniques
String Manipulation Techniques Image Gallery
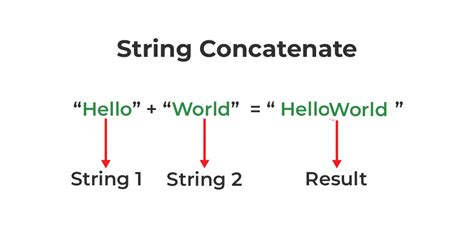
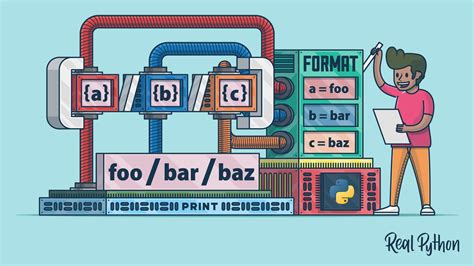
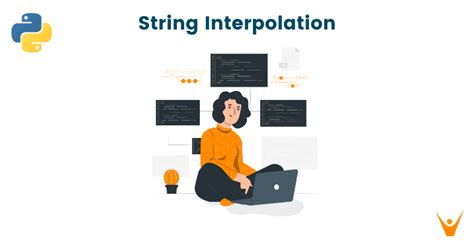
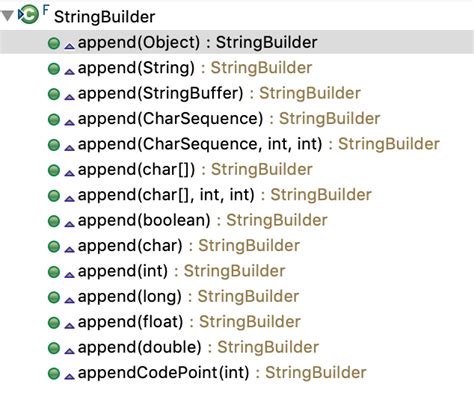
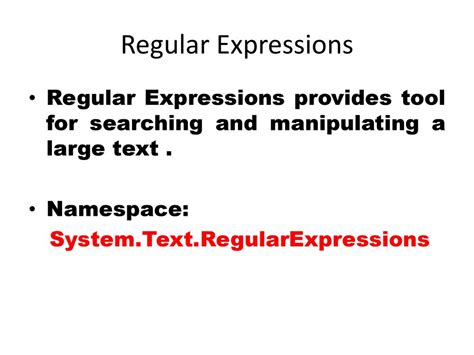
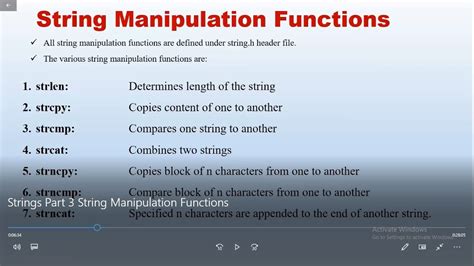
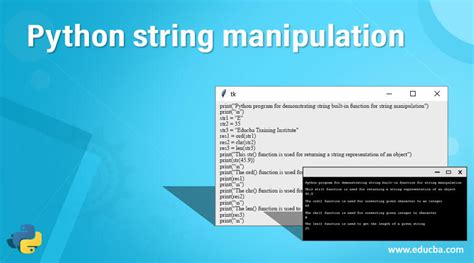
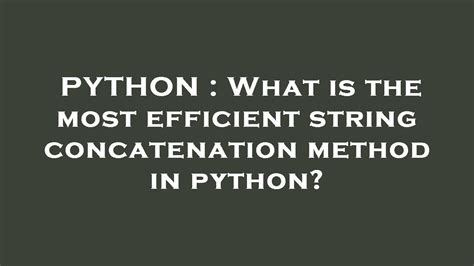
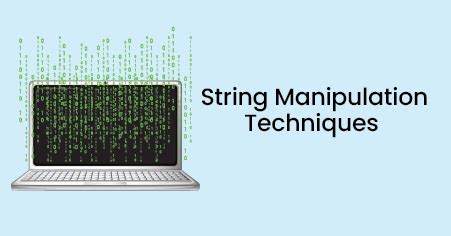
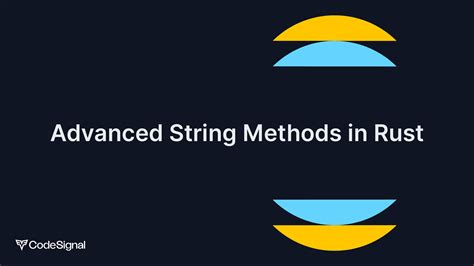
What is the most efficient way to append characters in a loop?
+
Using a StringBuilder or an equivalent class is generally the most efficient way to append characters in a loop, as it reduces the creation of temporary strings.
How do I append a character to a string using string formatting?
+
You can append a character to a string using string formatting by embedding the character within the format string, such as using f-strings in Python.
What are the benefits of using regular expressions for string manipulation?
+
Regular expressions offer powerful pattern matching capabilities, allowing for complex string manipulations, including appending characters under specific conditions, with great flexibility and precision.
What is the most efficient way to append characters in a loop?
+Using a StringBuilder or an equivalent class is generally the most efficient way to append characters in a loop, as it reduces the creation of temporary strings.
How do I append a character to a string using string formatting?
+You can append a character to a string using string formatting by embedding the character within the format string, such as using f-strings in Python.
What are the benefits of using regular expressions for string manipulation?
+Regular expressions offer powerful pattern matching capabilities, allowing for complex string manipulations, including appending characters under specific conditions, with great flexibility and precision.
In conclusion, appending characters to strings is a fundamental operation in programming and data processing, with various methods available depending on the context and programming language. By understanding and mastering these techniques, developers can improve their coding efficiency and versatility. Whether through concatenation operators, string formatting, StringBuilder classes, or regular expressions, the ability to manipulate strings effectively is crucial for a wide range of applications. We invite you to share your experiences or ask questions about string manipulation and character appending in the comments below, and to explore more topics related to programming and data processing.