Intro
Master VBA Right Function to extract strings. Learn syntax, examples, and uses in Excel VBA programming, including string manipulation and text processing techniques.
The VBA Right function is a powerful tool in Visual Basic for Applications (VBA) that allows users to extract a specified number of characters from the right side of a string. This function is particularly useful when working with text strings in Excel, Access, or other Microsoft Office applications that support VBA. In this article, we will delve into the details of the VBA Right function, including its syntax, examples, and practical applications.
The importance of string manipulation in VBA cannot be overstated. When working with data, it's common to encounter situations where you need to extract specific parts of a string, such as a date, a name, or a code. The Right function, along with its counterparts like Left and Mid, provides the flexibility to manipulate strings according to your needs. Understanding how to use these functions effectively can significantly enhance your ability to automate tasks and process data efficiently in VBA.
Before diving into the specifics of the Right function, it's worth noting that VBA offers a rich set of string functions that can be used in various combinations to achieve complex text manipulation tasks. The Right function, in particular, is straightforward to use and can be combined with other functions to extract, manipulate, and analyze text data.
Introduction to the VBA Right Function
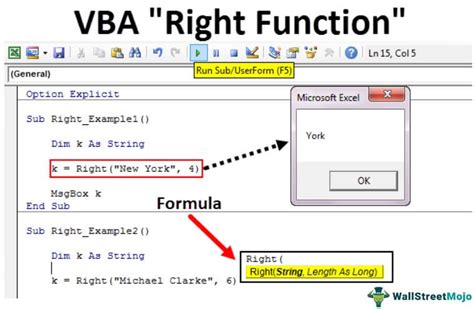
The VBA Right function is used to return a specified number of characters from the right side of a string. The syntax of the Right function is as follows:
Right(string, length)
Where:
string
is the string from which you want to extract characters.length
is the number of characters you want to extract from the right side of the string.
How to Use the Right Function
To use the Right function, you simply need to specify the string you're working with and the number of characters you want to extract from the right. For example, if you have a string "HelloWorld" and you want to extract the last 5 characters, you would use the following code: ``` MsgBox Right("HelloWorld", 5) ``` This would return "World".Practical Applications of the Right Function
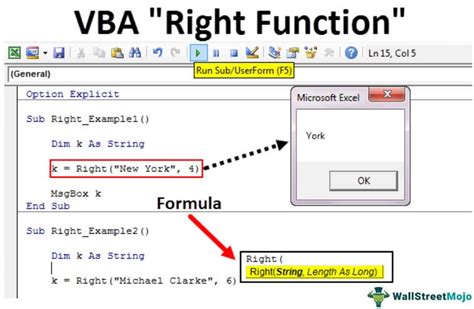
The Right function has numerous practical applications in VBA, especially when working with dates, codes, or any data that requires extraction of specific characters from the right. Here are a few examples:
- Extracting Year from a Date String: If you have a date string in the format "dd/mm/yyyy" and you want to extract the year, you can use the Right function.
- Extracting Extension from a File Name: When working with file names, you might need to extract the file extension. The Right function can be used in combination with the InStrRev function to achieve this.
- Data Analysis: In data analysis, you might encounter situations where you need to extract specific parts of a string for further analysis or processing.
Combining the Right Function with Other String Functions
The power of the Right function increases when it's combined with other VBA string functions. For instance, you can use the Left and Right functions together to extract a substring from a string, or you can use the Mid function to extract characters from the middle of a string.Here are some examples of combining the Right function with other string functions:
- Extracting a Substring:
Mid("HelloWorld", 3, 4)
extracts "lloW" from the string "HelloWorld". - Removing Characters: You can use the Replace function to remove specified characters from a string.
Common Errors and Troubleshooting
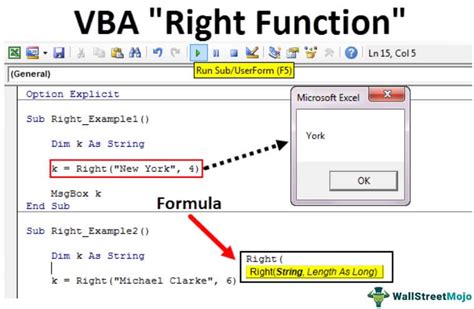
When working with the Right function, you might encounter a few common errors, such as:
- Error in Length Specification: If the length specified is greater than the length of the string, the function will return the entire string.
- Type Mismatch Error: Ensure that the arguments passed to the Right function are of the correct data type.
To troubleshoot these errors, it's essential to check the syntax of your code, verify the data types of the variables you're using, and ensure that the length you're specifying is valid for the given string.
Best Practices for Using the Right Function
To get the most out of the Right function and avoid common pitfalls, follow these best practices: - Always validate the input string to ensure it's not empty or null. - Verify that the length you're specifying is within the bounds of the string's length. - Use the Right function in combination with other string functions to achieve complex string manipulation tasks.Conclusion and Future Learning
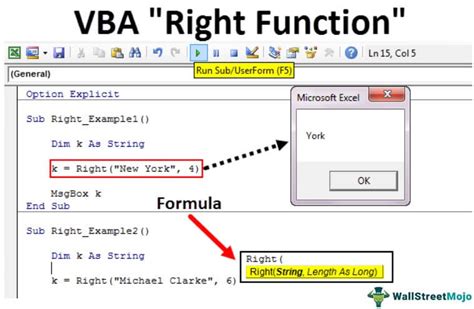
In conclusion, the VBA Right function is a versatile and powerful tool for extracting characters from the right side of a string. By understanding its syntax, practical applications, and how to combine it with other string functions, you can enhance your VBA programming skills and efficiently manipulate text data in your projects.
For future learning, consider exploring more advanced string manipulation techniques, such as using regular expressions or working with arrays of strings. Additionally, practicing with real-world examples and projects will help solidify your understanding of the Right function and other VBA concepts.
VBA Right Function Image Gallery
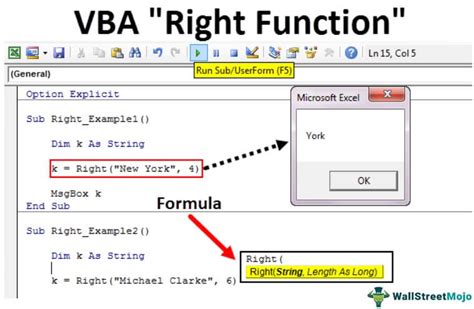
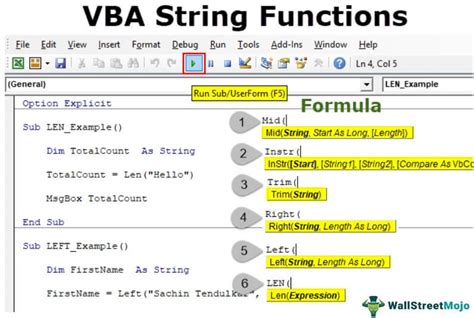
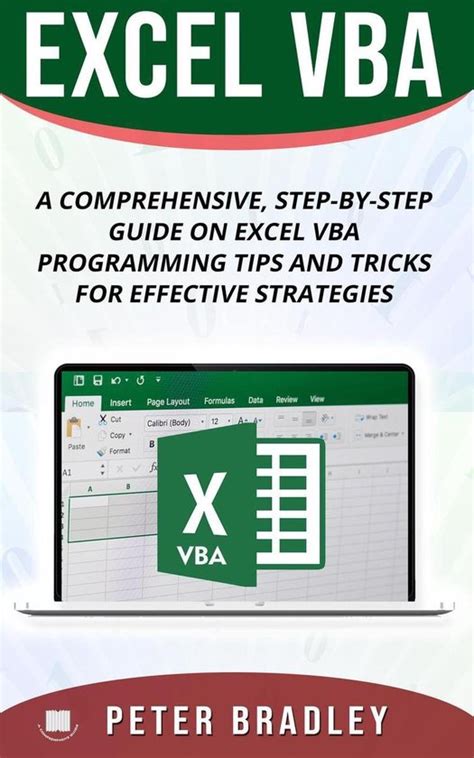
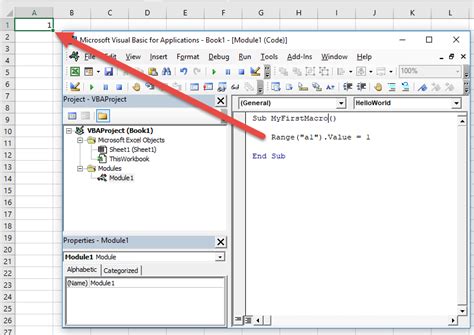
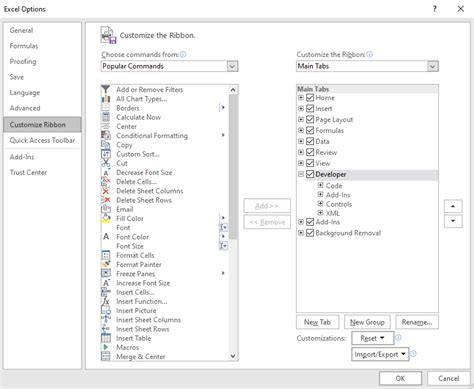
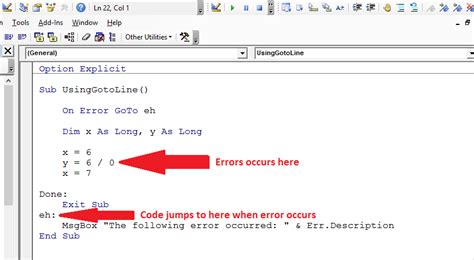
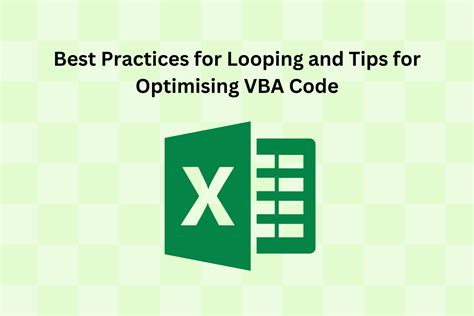
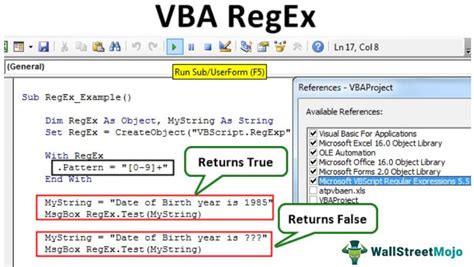
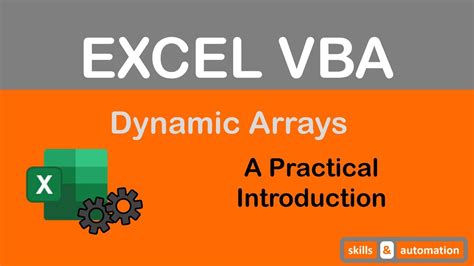
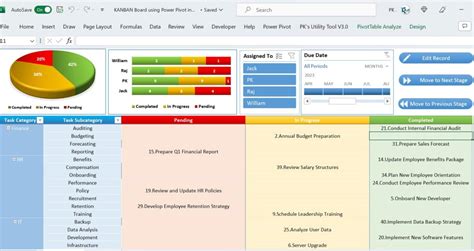
What is the VBA Right function used for?
+The VBA Right function is used to extract a specified number of characters from the right side of a string.
How do I use the Right function in VBA?
+To use the Right function, specify the string and the number of characters you want to extract from the right, like this: Right(string, length).
Can I combine the Right function with other string functions in VBA?
+
We hope this comprehensive guide to the VBA Right function has been informative and helpful. Whether you're a beginner or an advanced VBA user, understanding how to manipulate strings effectively can significantly enhance your productivity and the quality of your projects. Feel free to share your thoughts, ask questions, or provide tips on using the Right function in the comments below.