Intro
Checking for empty cells in Excel using VBA is a common task that can be accomplished in several ways, depending on what you need to achieve. Whether you're looking to identify empty cells, delete them, or perform some other operation, VBA provides the flexibility to handle such tasks efficiently. Below, we'll explore how to check for empty cells, including examples of how to identify them, delete rows containing empty cells, and more.
Checking for Empty Cells
To check if a cell is empty, you can use the IsEmpty
function or check the cell's value directly. Here's how you can do it:
Sub CheckEmptyCell()
If IsEmpty(Range("A1")) Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
' Alternatively
If Range("A1").Value = "" Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
End Sub
Looping Through Cells to Find Empty Ones
If you need to check multiple cells, you can loop through a range. This example checks each cell in column A and displays a message if an empty cell is found:
Sub FindEmptyCells()
Dim cell As Range
For Each cell In Range("A1:A100")
If IsEmpty(cell) Then
MsgBox "Cell " & cell.Address & " is empty"
End If
Next cell
End Sub
Deleting Rows with Empty Cells
You might need to delete rows that contain empty cells. Here's how you can do it:
Sub DeleteRowsWithEmptyCells()
Dim lastRow As Long
lastRow = Cells(Rows.Count, "A").End(xlUp).Row
Dim i As Long
For i = lastRow To 1 Step -1
If IsEmpty(Cells(i, 1)) Then
Rows(i).Delete
End If
Next i
End Sub
This script deletes any row in your worksheet where the cell in column A is empty, starting from the bottom to avoid issues with row indices changing as rows are deleted.
Checking for Empty Cells in a Specific Range
If you're working with a specific range and want to check for empty cells within that range, you can do so like this:
Sub CheckEmptyInRange()
Dim rng As Range
Set rng = Range("A1:E10") ' Define your range here
Dim cell As Range
For Each cell In rng
If IsEmpty(cell) Then
MsgBox "Cell " & cell.Address & " in the range is empty"
End If
Next cell
End Sub
Practical Example: Highlighting Empty Cells
Sometimes, visually identifying empty cells can be helpful. You can use the following code to highlight empty cells in a specified range:
Sub HighlightEmptyCells()
Dim rng As Range
Set rng = Range("A1:E10") ' Define your range here
Dim cell As Range
For Each cell In rng
If IsEmpty(cell) Then
cell.Interior.Color = vbYellow ' Highlights empty cells in yellow
End If
Next cell
End Sub
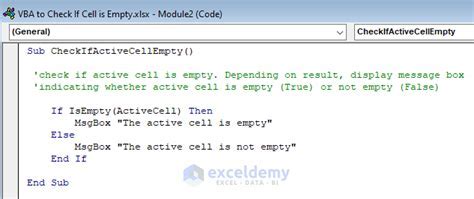
Gallery of VBA Examples
VBA Examples Image Gallery
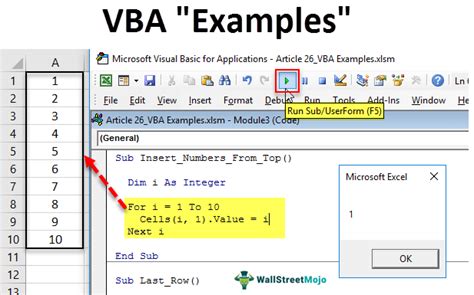
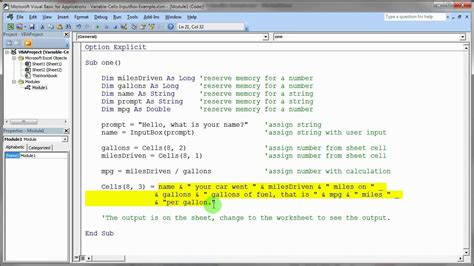
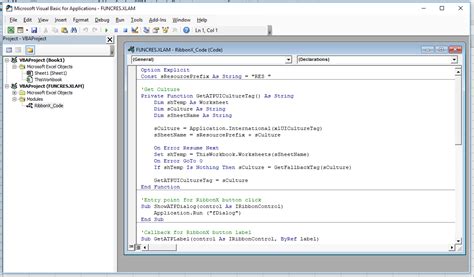
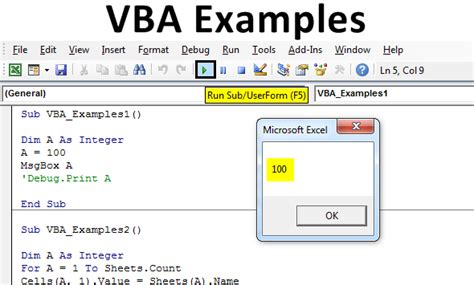
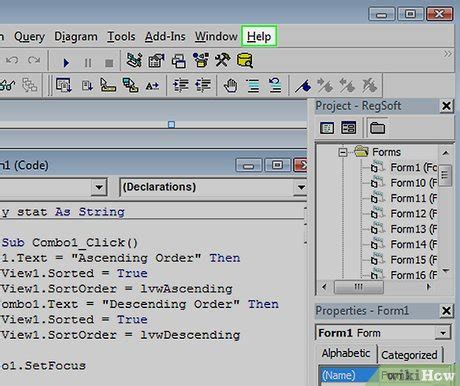
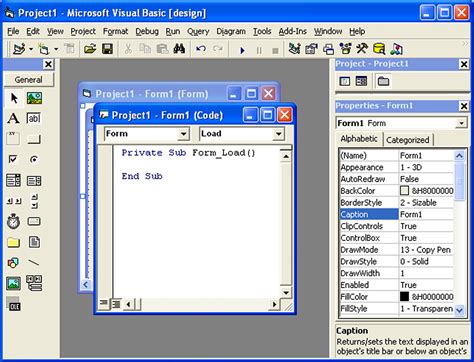
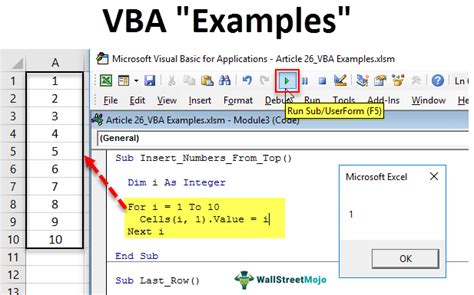
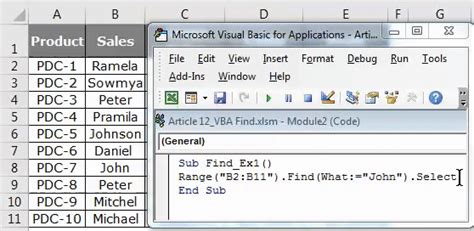
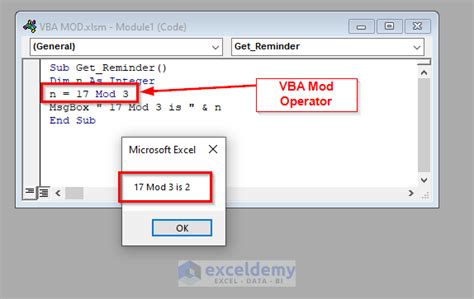
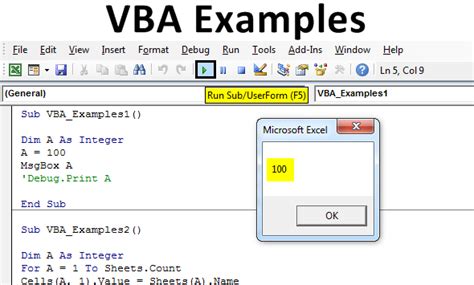
FAQs
What is VBA used for in Excel?
+VBA (Visual Basic for Applications) is used for creating and automating tasks in Excel, such as formatting, data manipulation, and more.
How do I open VBA in Excel?
+You can open VBA in Excel by pressing Alt + F11 or navigating to Developer > Visual Basic.
Can I use VBA to automatically delete empty rows?
+In conclusion, checking for empty cells in Excel using VBA is a versatile and powerful tool that can simplify many tasks, from data cleaning to automation. Whether you're a beginner looking to understand the basics or an advanced user seeking to optimize your workflows, mastering VBA can significantly enhance your productivity with Excel. Feel free to share your experiences or ask questions about using VBA for handling empty cells or other tasks in the comments below.